Using Moment.js in ServiceNow
Start using world famous library for date manipulations
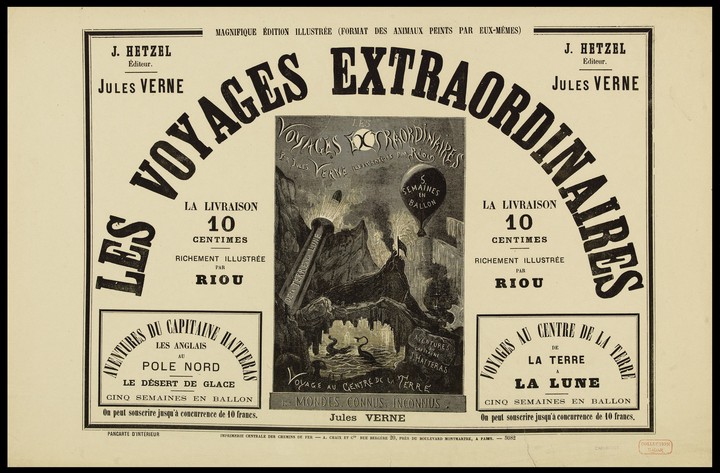
Moment.js is great library for interacting with date and time. It helps you parse, validate, manipulate, and display dates.
Did not hear about Moment.js? Check the docs.
How to install for server side usage
- Download the source code at https://momentjs.com/downloads/moment.js
- Create a script include named “moment” and fill with source code.
- The
moment
object is ready to be used in all server side code expressions.
How to install for client side usage
If you want to use Moment.js within Service Portal - it’s already there OOB. Just try using the
moment
object from a client script.
- Download the source code at https://momentjs.com/downloads/moment.js
- Create a UI Script named “moment”: Activate “Global” checkbox, copy source code to the “Script” field.
- The
moment
object is ready to be used in all server side code expressions.
Examples for server side
For instance, we are editing a business rule.
var sncDateFormat = "YYYY-MM-DD hh:mm:ss";
var glideDateA = new GlideDateTime();
var momentDateA = moment(glideDateA, sncDateFormat);
gs.info(momentDateA.format()); // Default Moment.js format (ISO 8601)
/**
// Validate if Date is BEFORE or AFTER a date
**/
// Create a momentjs object with a date, that is 5 days from actual
var momentDateB = moment(momentDateA).add(5, "days");
// Compare dates
gs.info(momentDateB.isBefore(momentDateA)); // should be FALSE
gs.info(momentDateB.isAfter(momentDateA)); // should be TRUE
/**
// Validate if between dates
**/
// Clone the moment date object and add 2 days
var momentDateC = moment(momentDateA).add(2, "days");
// Then find out if the date is betweeen another two dates
gs.info(momentDateC.isBetween(momentDateA, momentDateB)); // Should be TRUE
/**
// Modify date field and write back to a record
// Example for a business rule:
**/
var sncDateFormat = "YYYY-MM-DD hh:mm:ss";
var startDate = current.getValue("start_date");
var momentDate = moment(startDate, sncDateFormat).add(1, "days");
current.start_date = new GlideDateTime(momentDate.format(sncDateFormat));
Examples for client side
Using Moment.js in a client script would be like:
/**
* This onSubmit function validates of the date chosen for variable "Valid From"
* is before the date chosen in the variable "Valid To"
*/
function onSubmit() {
// Get user date format
var userDateFormat = getUserDateFotmatForMomentjs();
// 1. Create Momentjs objects out of variable values
var validFrom = moment(g_form.getValue("valid_from"), userDateFormat);
var validTo = moment(g_form.getValue("valid_to"), userDateFormat);
// 2. Validate if Valid From "is before" Valid To
if (!validFrom.isBefore(validTo)) {
g_form.addErrorMessage("Valid To should be after Valid From");
return false;
}
// 3. Check that Valid To is not more than 12 month in the past
if (
g_form.getValue("user_type") == "UAT User" &&
validFrom.add("12", "month").isBefore(validTo)
) {
g_form.addErrorMessage("Valid To cannot be more than 12 month in future");
return false;
}
}
/**
* Construct a proper date format for momentjs
* to be able to use it like:
* moment(g_form.getValue('date), userDateFormat)
*/
function getUserDateFotmatForMomentjs() {
var dateFormat = g_user_date_format.toUpperCase();
var dateTimeFormat = g_user_date_time_format;
return dateFormat + dateTimeFormat.substring(dateFormat.length);
}